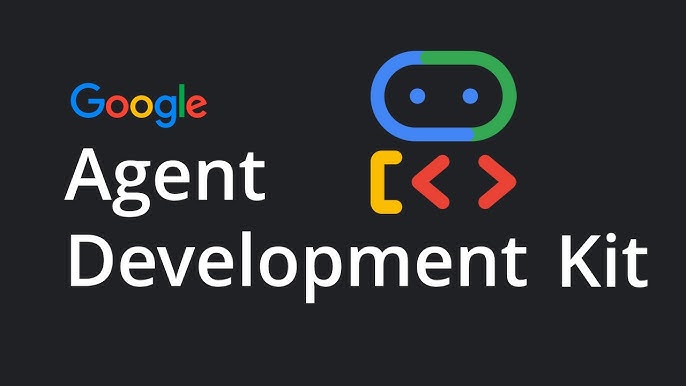
Google ADK: Build AI Agents with Real-Time Data & Multi-Agent Systems
Discover how Google’s Agent Development Kit (ADK) enables developers to build AI agents with real-time data grounding, multi-agent collaboration, and enterprise scalability. Includes code examples, Mermaid diagrams, and a supply chain case study.
Google’s Agent Development Kit (ADK): The Ultimate Guide to Building Enterprise-Ready AI Agents #
🚀 Introduction: Why Google ADK is a Game-Changer #
Imagine AI agents that:
✅ Access real-time Google Maps traffic data for logistics optimization.
✅ Collaborate in teams (e.g., inventory + routing agents) via Agent-to-Agent (A2A) protocols.
✅ Enforce hard-coded business rules (e.g., "Never exceed 500-mile delivery routes").
Google’s Agent Development Kit (ADK) makes this possible—a framework for building enterprise-grade AI agents with:
- Live data grounding (Google Maps, Search, ERP systems).
- Deterministic control (constraints + LLMs).
- Multi-agent orchestration at scale.
💡 Think of ADK as the "Android OS for AI agents"—standardized, scalable, and ecosystem-integrated.
🔧 What Makes ADK Unique? (vs. LangChain, AutoGen, n8n) #
Comparison Table #
Feature | Google ADK | LangChain | AutoGen | n8n |
---|---|---|---|---|
Real-Time Data | ✅ Native Maps/Search | ❌ Manual RAG | ❌ | ⚠️ Limited |
Multi-Agent | ✅ A2A Protocol | ✅ LangGraph | ✅ Teams | ❌ |
Enterprise Security | ✅ IAM, VPC-SC | ❌ Self-managed | ❌ | ✅ |
Hard Constraints | ✅ Policy enforcement | ❌ LLM-only | ⚠️ Limited | ❌ |
Deployment | ✅ Vertex AI, GKE | ❌ Self-hosted | ❌ | ✅ Cloud |
When to Use ADK? #
- ✅ Ideal for:
- Real-time logistics (e.g., live traffic + inventory agents).
- Enterprise multi-agent workflows (e.g., customer service + ERP integration).
- ⚠️ Alternatives:
- Simple chatbots → LangChain.
- Quick prototypes → AutoGen.
- API automation → n8n.
🏗️ Building a Supply Chain Agent with ADK: Step-by-Step #
Case Study: Vaccine Distribution with Multi-Agent Teams #
Problem: Distribute COVID vaccines under:
- Temperature control (2-8°C).
- Expiration date prioritization.
- Real-time traffic/weather updates.
Architecture Diagram #
graph TD
A[User Request] --> B(Orchestrator Agent)
B --> C[Data Collector Agent]
B --> D[Route Optimizer Agent]
B --> E[Inventory Agent]
C --> F[Google Maps API]
C --> G[Weather API]
D --> H[Google OR-Tools]
E --> I[SAP ERP]
B --> J[Report Generator Agent]
Agents & Roles #
Agent | Role | Tools | Output |
---|---|---|---|
Data Collector | Fetches traffic/weather | Maps API | JSON data |
Route Optimizer | Calculates cold-compliant routes | OR-Tools | Optimized routes |
Inventory Agent | Tracks expiring batches | SAP ERP | Stock levels |
Code Implementation #
from google.adk import Agent, GroundingSource
# Initialize with Google Maps grounding
maps_grounding = GroundingSource(
name="google_maps",
api_key=os.getenv("MAPS_API_KEY")
)
# Define vaccine distribution agent
vaccine_agent = Agent(
role="Cold Chain Manager",
grounding_sources=[maps_grounding],
constraints=[
"temperature_2_8c", # Hard-coded rule
"prioritize_expiring_batches"
],
tools=["or_tools", "sap_erp"]
)
# Optimize delivery routes
response = vaccine_agent.run(
"Optimize delivery for 50 hospitals in Texas"
)
⚡ Advanced Features: Dynamic Agents & Cross-Agent Learning #
1. Dynamic Agent Spawning #
Create agents on-demand for edge cases (e.g., hurricanes):
def spawn_emergency_agent(incident_type):
return Agent(
role=f"{incident_type} Responder",
grounding_sources=[maps_grounding, weather_grounding],
lifetime="ephemeral" # Terminates post-task
)
if hurricane_alert:
hurricane_agent = spawn_emergency_agent("Hurricane")
crew.add_agent(hurricane_agent)
2. Shared Knowledge Graph #
Agents learn from collective history:
knowledge_graph = ADKKnowledgeGraph(
sources=["supply_chain_decisions", "route_history"],
sync_frequency="5m"
)
route_optimizer.learn_from(
knowledge_graph.query("past_optimizations?region=northeast")
)
📊 ADK vs. Competitors: Technical Deep Dive #
1. Data Grounding: ADK vs. LangChain #
ADK (1 line):
response = adk.query("Nearest warehouse", ground_with="google_maps")
LangChain (Manual):
retriever = GoogleSearchAPIWrapper() + VectorstoreIndexCreator()
2. Multi-Agent Communication #
- ADK’s A2A Protocol > AutoGen’s Custom Teams
- Standardized handoffs (e.g., logistics → inventory agents).
3. Enterprise Security #
- ADK: Pre-integrated with VPC-SC, IAM, MCP.
- Others: Self-managed (risky for HIPAA/GDPR).
🚀 Getting Started with ADK #
Step 1: Install & Authenticate #
pip install google-adk
gcloud services enable adk.googleapis.com
Step 2: Build Your First Agent #
from google.adk import Agent
agent = Agent(
grounding_sources=["google_search"],
llm="gemini-pro"
)
response = agent.run("Stock price of GOOG")
print(response.grounded_content) # Verified live data
Step 3: Deploy to Vertex AI #
from google.cloud import adk_deploy
adk_deploy.to_vertex(
agent,
project="my-project",
region="us-central1"
)
🎯 Key Takeaways #
- ADK excels at:
- Real-time data agents (Maps, Search, ERP).
- Multi-agent systems (A2A protocol).
- Enterprises using Google Cloud.
- Alternatives better for:
- Simple chatbots → LangChain.
- Prototyping → AutoGen.
- API workflows → n8n.
📌 Next Steps:
Sources: