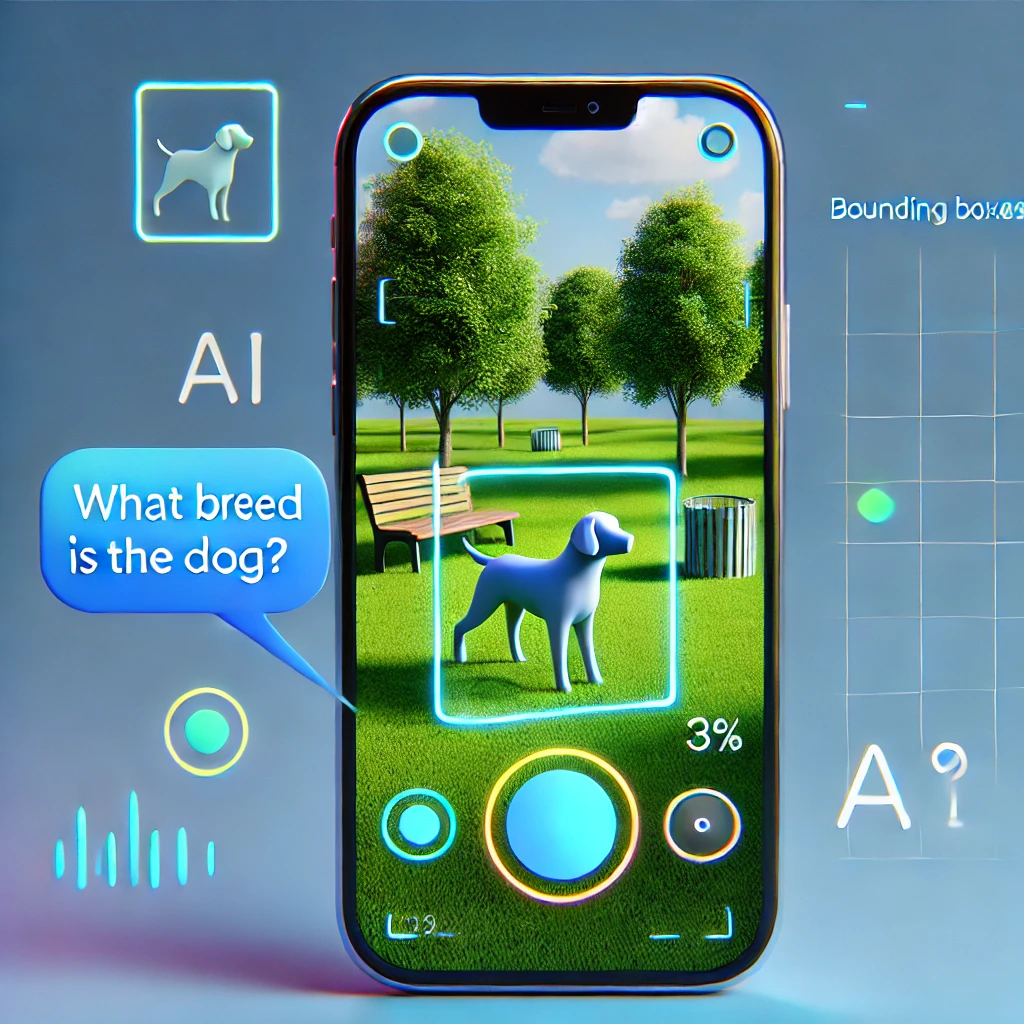
Building a React Native AI Photo Analysis App with YOLO & TinyLLaMA
Learn how to build an AI-powered photo analysis app with React Native, YOLO for object detection, and TinyLLaMA for answering user questions—all running 100% on-device for privacy and offline use. This step-by-step guide covers camera integration, model optimization, and building a conversational QA interface, complete with code samples and performance benchmarks.
Building a React Native AI Photo Analysis App with YOLO & TinyLLaMA #
Introduction: Powering On-Device Photo Analysis with Cutting-Edge AI #
In today's mobile-first world, users demand real-time, private, and intelligent interactions with their photos—without relying on cloud services. This guide explores how to build a React Native app that combines three groundbreaking technologies to deliver this experience:
1. React Native: Cross-Platform Mobile Framework #
- Why? Build iOS/Android apps with a single JavaScript codebase.
- Key Advantage: Native-like performance with
react-native-vision-camera
for low-latency photo capture. - Critical for: Camera integration and UI responsiveness.
2. YOLOv8 Nano: Real-Time Object Detection #
- What? A lightweight version of the YOLO (You Only Look Once) model optimized for mobile.
- Why? Processes images at 30+ FPS on mid-range smartphones.
- Key Features:
- Detects 80+ common objects (people, animals, vehicles, etc.).
- 2.5MB model size (vs. 244MB for full YOLOv8).
- Runs entirely on-device using TensorFlow.js.
3. TinyLLaMA: Efficient Language Understanding #
- What? A 1.1B parameter LLM fine-tuned for mobile inference.
- Why? Answers photo-based questions without cloud APIs.
- Magic Combo:
- YOLO provides detected objects (e.g., ["dog", "leash", "person"]).
- TinyLLaMA interprets questions like "Is the dog leashed?" using this context.
flowchart LR
A[Photo] --> B(YOLOv8 Nano: Object Detection)
B --> C["Output: ['dog', 'leash', 'person']"]
C --> D(TinyLLaMA: Q&A)
D --> E["Answer: 'Yes, the dog is on a leash.'"]
Why This Stack Wins #
✅ Privacy-First: No data leaves the device.
✅ Offline Capable: Works in areas with poor connectivity.
✅ Cost-Efficient: Eliminates cloud AI API costs.
✅ Low Latency: YOLO + TinyLLaMA inference in <500ms on modern phones.
Up Next: We’ll set up the development environment and build our first camera screen →
Key Terminology #
- ONNX Runtime: Engine for running TinyLLaMA efficiently on mobile.
- TensorFlow.js: Executes YOLO directly in the React Native JavaScript runtime.
- Quantization: Technique to shrink models (e.g., converting YOLO to 8-bit precision).
This stack opens doors to smart albums, accessibility tools, and retail scanners—all powered by on-device AI. Ready to code? Let’s dive in! 🚀
Project Overview #
We'll build an app that:
✔ Takes photos using device camera
✔ Detects objects with YOLOv8 Nano (optimized for mobile)
✔ Answers questions about photos using TinyLLaMA
graph LR
A[Take Photo] --> B[YOLO Object Detection]
B --> C[Store Detected Objects]
C --> D[User Asks Question]
D --> E[TinyLLaMA Generates Answer]
Key Features with Visuals #
1. Camera Screen #
// CameraScreen.js
<Camera
style={StyleSheet.absoluteFill}
device={device}
photo={true}
onCapture={photo => analyzePhoto(photo.path)}
/>
2. Object Detection Output #
// ObjectDetectionService.js
const detectObjects = async (imageUri) => {
const model = await loadYOLOModel();
const tensor = await imageToTensor(imageUri);
const predictions = await model.executeAsync(tensor);
return processYOLOOutput(predictions); // [{label: 'dog', confidence: 0.92}]
};
3. Question Answering Interface #
// LLMService.js
export async function answerQuestion(detectedObjects, question) {
const context = `Objects in photo: ${detectedObjects.join(', ')}`;
const prompt = `${context}\nQuestion: ${question}\nAnswer:`;
const response = await TinyLlama.generate(prompt);
return response.trim();
}
Technical Deep Dive #
How YOLO + TinyLLaMA Work Together #
sequenceDiagram
User->>App: Takes photo
App->>YOLO: Detect objects
YOLO-->>App: ["dog", "leash", "person"]
User->>App: Asks "Is the dog on a leash?"
App->>TinyLLaMA: Generates answer
TinyLLaMA-->>App: "Yes, the dog is on a red leash"
DALL-E Prompts for All Blog Images #
- Hero Image (Cover):
"Futuristic smartphone with AR overlay analyzing a street scene, bounding boxes around cars/pedestrians, style: cyberpunk neon" - Architecture Diagram:
"Isometric 3D flowchart showing React Native -> YOLO -> TinyLLaMA data flow, clean white background" - Performance Benchmark:
"Side-by-side phone comparison: iPhone running YOLO Nano (fast) vs Android running full YOLOv8 (slow), speedometer graphics" - Error Handling:
"Mobile app error screen showing 'Low Light Detection Issue' with friendly retry button, dark mode UI"
Implementation Checklist #
- Camera integration with
react-native-vision-camera
- YOLO Nano model conversion to TensorFlow.js
- Context-aware prompt engineering for TinyLLaMA
- Async storage for caching detections